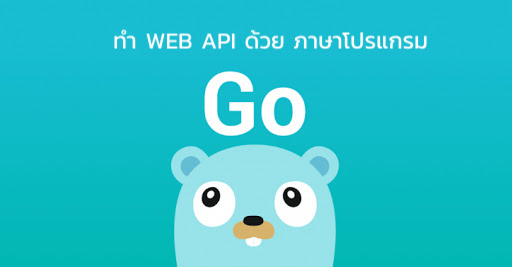
บทเรียนการสร้าง API ด้วยภาษา Go สำหรับผู้เริ่มต้นในการพัฒนา Web API เพื่อทำส่วนต่อประสานโปรแกรมเบื้องต้นโดยใช้ package ของ net-http
บทเรียนก่อนหน้า : รวมบทเรียนภาษา go
เริ่มต้นสร้าง Package ของเราขึ้นมา ชื่อ golang แล้วสร้าง directory สำหรับทำ api ขึ้นมาชื่อว่า “api” โดยรันคำสั่งต่อไปนี้:
$ mkdir api $ cd api
เปิด Folder API ขึ้นมาสร้างไฟล์ index.go แล้วเขียนคำสั่งต่อไปนี้:
package main import ( "fmt" ) func main() { fmt.Println("Test API") }
ลองรันคำสั่งทดสอบก่อนโดยเปิด Terminal ขึ้นมาพิมพ์ว่า:
$ go run index.go
ถ้ามี Console รันคำสั่ง PrintIn ก็โอเคครับ
ต่อมาทำการ Import Package สำหรับจัดการเว็บ net-http และ json กันหน่อยโดยเพิ่ม
"encoding/json" "net/http"
โดยการประกาศเพิ่มดังนี้:
import ( "encoding/json" "fmt" "net/http" )
ทำการสร้าง โครงสร้างข้อมูล Struct ขึ้นมาเพื่อนำมาแสดงผลผ่าน JSON ในรูปแบบ Key-Value ชื่อว่า userData
type userData struct { Userid int Firstname string Lastname string Email string }
ข้อสังเกต Key ของ Struct ในภาษา Go จำเป็นต้องขึ้นต้นด้วย Capital หรืออักษรตัวใหญ่, ดังนั้นเราต้องเอา Struct ของ userData มาทำเป็นข้อมูล value ผ่านฟังก์ชันใหม่คือ getUsers ดังนี้:
func getUsers(w http.ResponseWriter, r *http.Request) { //(2) userResponse := userData{ Userid: 28566777, Firstname: "Banyapon", Lastname: "Poolsawas", Email: "[email protected]", } json.NewEncoder(w).Encode(userResponse) }
ใช้ Package “encoding/json” เข้ามาช่วยแปลง Struct เป็น JSON โดยอัดข้อมูลเข้าไปโดยตรงซึ่งเป็นข้อมูลปลอม ยัดเข้าไปใน userResponse โดยตรงเพื่อทำการ json Encoder
สร้างฟังก์ชันใหม่ชื่อว่า handleRequest()
func handleRequest() { http.HandleFunc("/", users) http.HandleFunc("/users", getUsers) http.ListenAndServe(":8080", nil) }
กลับไปที่ func Main( ) ให้เปลี่ยนการเรียกคำสั่งเป็น:
func main() { //fmt.Println("Test API") handleRequest() }
ภาพรวม code ของ index.go จะเป็นดังนี้:
package main import ( "encoding/json" "fmt" "net/http" ) type userData struct { Userid int Firstname string Lastname string Email string } func getUsers(w http.ResponseWriter, r *http.Request) { userResponse := userData{ Userid: 28566777, Firstname: "Banyapon", Lastname: "Poolsawas", Email: "[email protected]", } json.NewEncoder(w).Encode(userResponse) } func users(w http.ResponseWriter, r *http.Request) { fmt.Fprint(w, "success") } func handleRequest() { http.HandleFunc("/", users) http.HandleFunc("/users", getUsers) http.ListenAndServe(":8080", nil) } func main() { //fmt.Println("Test API") handleRequest() }
ทำการรันคำสั่งใน Terminal แล้วทดสอบที่ http://localhost:8080/users
ผลลัพธ์: